Ruby Word and Letter Counter
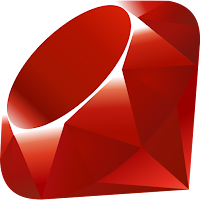
Ruby Word and Letter Counter This simple Program will accept a string and return the total number of words and show the number of occurrences for each letter. Ruby #Enter a string to see word & letter counts puts "Enter your text:" text = gets.chomp puts "#{text}" word_count = text.split(' ').count puts "Word count is #{word_count} \nLetter Frequency is:" text.downcase! freqs = {} freqs.default = 0 text.each_char { |char| freqs[char] += 1} ("a".."z").each {|x| puts "#{x} : #{freqs[x]}" } puts "Thank You" Click here to run this code More Free Code Examples: Javascript Substitution Cipher Javascript Easy Battleship Game Hello World in 10 Programming Languages Try these Fun Games by Bobbie: Travel Blast Game New York Play and Learn Russian Battlestarship Game Take a look at these Groovy Codes: